
c 在接受输入时怎么做到不中断程序
在C语言中,实现接受输入时不中断程序的运行,可以采用多种方法,以下是几种常见的实现方式:
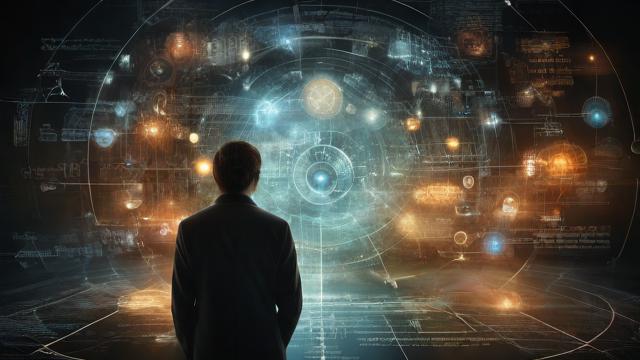
1、使用非阻塞I/O
原理:通过设置文件描述符为非阻塞模式,使得read函数在没有数据可读时立即返回,而不是阻塞程序。
示例代码
#include <stdio.h> #include <stdlib.h> #include <unistd.h> #include <fcntl.h> int main() { int flags = fcntl(STDIN_FILENO, F_GETFL, 0); if (flags == 1) { perror("fcntl"); exit(EXIT_FAILURE); } flags |= O_NONBLOCK; if (fcntl(STDIN_FILENO, F_SETFL, flags) == 1) { perror("fcntl"); exit(EXIT_FAILURE); } printf("Please enter something within 5 seconds: "); char buffer[128]; ssize_t nread; int count = 0; while (count < 5) { nread = read(STDIN_FILENO, buffer, sizeof(buffer)); if (nread > 0) { buffer[nread] = '\0'; printf("You entered: %s ", buffer); break; } else if (nread == 1 && errno != EAGAIN) { perror("read"); exit(EXIT_FAILURE); } // 模拟其它工作 sleep(1); count++; } if (count == 5) { printf("No input, doing other work... "); } return 0; }
注意事项:需要对fcntl
、read
等函数的返回值进行检查,并根据具体错误码进行相应处理,不同平台对非阻塞I/O的支持和实现可能略有差异,需要进行跨平台兼容性测试。
2、使用多线程
原理:创建一个专门的线程用于处理输入,主线程则继续执行其他任务,这样可以避免主线程因等待输入而被阻塞。
示例代码
#include <stdio.h> #include <stdlib.h> #include <unistd.h> #include <pthread.h> void* input_thread(void* arg) { char buffer[100]; while (1) { if (fgets(buffer, sizeof(buffer), stdin) != NULL) { printf("Input: %s ", buffer); } } return NULL; } int main() { pthread_t tid; pthread_create(&tid, NULL, input_thread, NULL); while (1) { printf("Doing other work... "); sleep(1); // 模拟其它工作 } pthread_join(tid, NULL); return 0; }
注意事项:需要注意线程的创建、同步和资源管理等问题,要确保共享资源的访问是线程安全的,避免出现数据竞争和死锁等情况。
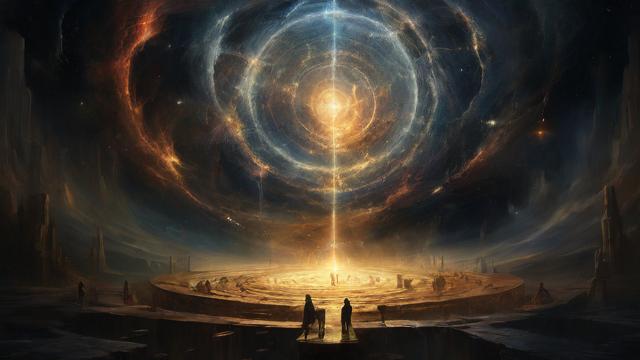
3、使用select函数实现超时机制
原理:利用select函数监控多个文件描述符的状态,包括标准输入,通过设置超时时间,如果在规定时间内没有输入数据,select函数将返回,程序可以根据返回值判断是否超时,并执行相应的操作。
示例代码
#include <stdio.h> #include <stdlib.h> #include <unistd.h> #include <sys/time.h> #include <sys/types.h> int main() { struct timeval tv; fd_set readfds; int retval; tv.tv_sec = 5; tv.tv_usec = 0; FD_ZERO(&readfds); FD_SET(STDIN_FILENO, &readfds); retval = select(1, &readfds, NULL, NULL, &tv); if (retval == 1) { perror("select()"); exit(EXIT_FAILURE); } else if (retval) { printf("Data is available now. "); char buffer[128]; read(STDIN_FILENO, buffer, sizeof(buffer)); printf("You entered: %s ", buffer); } else { printf("No data within five seconds. "); } return 0; }
注意事项:需要正确设置select函数的参数,包括文件描述符集、超时时间等,要对select函数的返回值进行准确判断,以确定是有数据可读、发生错误还是超时。
4、使用poll函数实现超时机制
原理:与select函数类似,poll函数也可以监控多个文件描述符的状态,并且可以通过设置超时时间来控制等待时间,当超时时,poll函数会返回,程序可以根据返回值决定后续的操作。
示例代码
#include <stdio.h> #include <stdlib.h> #include <unistd.h> #include <poll.h> int main() { struct pollfd pfd; int ret; char buffer[128]; pfd.fd = STDIN_FILENO; pfd.events = POLLIN; while (1) { ret = poll(&pfd, 1, 5000); // 超时时间为5000毫秒(5秒) if (ret == 1) { perror("poll"); exit(EXIT_FAILURE); } else if (ret) { if (pfd.revents & POLLIN) { read(STDIN_FILENO, buffer, sizeof(buffer)); printf("You entered: %s ", buffer); break; } } else { printf("No input within 5 seconds, doing other work... "); } } return 0; }
注意事项:需要正确初始化pollfd结构体,并设置合适的事件和超时时间,同样,要根据poll函数的返回值进行正确的处理,以实现预期的功能。
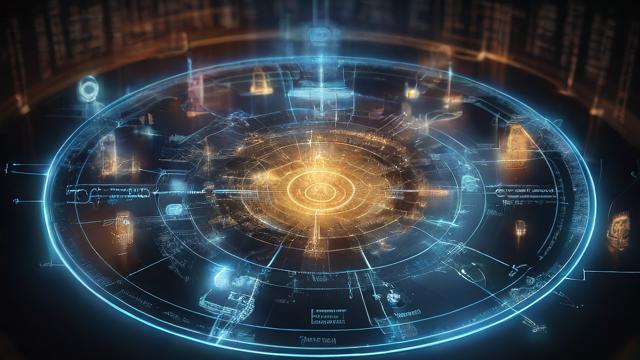
这些方法各有优缺点,选择哪种方法取决于具体的应用场景和需求,在实际编程中,需要根据具体情况选择合适的方法来实现接受输入时不中断程序的功能。
作者:豆面本文地址:https://www.jerry.net.cn/articals/35681.html发布于 2025-02-27 14:51:07
文章转载或复制请以超链接形式并注明出处杰瑞科技发展有限公司